오늘의 노래!!!
Cupid
- 아티스트
- FIFTY FIFTY
- 앨범
- The Beginning: Cupid
- 발매일
- 2023.02.24
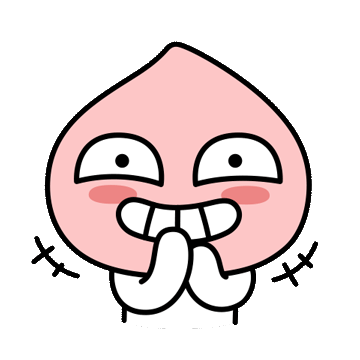
잠깐 게임으로 머리 식히기~~
[0] 핀볼게임
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta name="author" content="bro">
<meta name="date" content="">
<meta name="description" content="">
<link rel="stylesheet" href="style.css">
<link rel="shortcut icon" href="favicon.ico?=v2" type="image/x-icon">
<title></title>
<style>
canvas{
background-color: whitesmoke;
display: block;
margin: auto;
}
</style>
</head>
<body>
<canvas id="can" width="480" height="320"></canvas>
</body>
<script>
const canvas = document.getElementById("can");
const ctx = canvas.getContext("2d");
const ballRadius = 10;
let x = canvas.width/2;
let y = canvas.height - 30;
let dx = 2;
let dy = -2;
const paddleWidth= 75;
const paddleHeight= 30;
let paddleX = (canvas.width/2 - paddleWidth)/2;
let paddleY = canvas.height - 15;
let rightPressed = false;
let leftPressed = false;
let scorenum = 0;
let lifeNum = 3;
// key이벤트 추가
document.addEventListener("keydown", keydownHandler, false);
document.addEventListener("keyup", keyupHandler, false);
function keydownHandler(e){
if(e.keyCode == 39){
rightPressed = true;
} else if (e.keyCode == 37){
leftPressed = true;
}
}
function keyupHandler(e){
if(e.keyCode == 39){
rightPressed = false;
} else if (e.keyCode == 37){
leftPressed = false;
}
}
function life(){
ctx.font="16px Arial";
ctx.fillStyle="blue";
ctx.fillText("Life : " + lifeNum, 420,20);
}
function score(){
ctx.font = "16px Arial";
ctx.fillStyle = "red";
ctx.fillText("score :"+scorenum, 8,20);
scorenum++;
}
function paddle(){
ctx.beginPath();
ctx.rect(paddleX, paddleY, paddleWidth, paddleHeight);
ctx.fillStyle = "orange";
ctx.fill();
ctx.closePath();
}
function ball(){
ctx.beginPath();
// arc(원의 중심의 x좌표, y좌표, 원의반지름, 시작각, 끝각, 그리는 방향);
ctx.arc(x, y, ballRadius, 0, Math.PI*2);
ctx.fillStyle = "red";
ctx.fill();
ctx.strokeStyle = "black";
ctx.stroke();
ctx.closePath();
};
function draw(){
ctx.clearRect(0, 0, canvas.width, canvas.height);
ball();
paddle();
score();
life();
if(x >= canvas.width - ballRadius || x <= ballRadius){
dx = -dx;
}
if(y <= ballRadius){
dy = -dy;
} else if( y >= paddleY - ballRadius){
if(x > paddleX && x < paddleX + paddleWidth){
dy = - dy;
}
if(y >= canvas.height - ballRadius){
lifeNum --;
}else{
alert('게임종료\n 현재점수 : '+scorenum);
}
}
if(rightPressed && paddleX <= canvas.width - paddleWidth){
paddleX +=7;
}
if(leftPressed && paddleX >=0){
paddleX -=7;
}
x += dx;
y += dy;
}
setInterval(draw, 10);
</script>
</html>
[1] 빙고게임
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
<style>
table{
margin: auto;
}
td{
width: 100;
height: 100px;
border: 5px solid powderblue;
font-size: 15px;
text-align: center;
}
</style>
</head>
<body>
<table width="500" height="500">
<tr>
<td id="1" onclick="colorChange(this, 1)">1</td>
<td id="2" onclick="colorChange(this, 2)">3</td>
<td id="3" onclick="colorChange(this, 3)">5</td>
<td id="4" onclick="colorChange(this, 4)">7</td>
<td id="5" onclick="colorChange(this, 5)">9</td>
</tr>
<tr>
<td id="6" onclick="colorChange(this, 6)">11</td>
<td id="7" onclick="colorChange(this, 7)">13</td>
<td id="8" onclick="colorChange(this, 8)">15</td>
<td id="9" onclick="colorChange(this, 9)">17</td>
<td id="10" onclick="colorChange(this, 10)">19</td>
</tr>
<tr>
<td id="11" onclick="colorChange(this, 11)">21</td>
<td id="12" onclick="colorChange(this, 12)">23</td>
<td id="13" onclick="colorChange(this, 13)">25</td>
<td id="14" onclick="colorChange(this, 14)">27</td>
<td id="15" onclick="colorChange(this, 15)">29</td>
</tr>
<tr>
<td id="16" onclick="colorChange(this, 16)">31</td>
<td id="17" onclick="colorChange(this, 17)">33</td>
<td id="18" onclick="colorChange(this, 18)">35</td>
<td id="19" onclick="colorChange(this, 19)">37</td>
<td id="20" onclick="colorChange(this, 20)">39</td>
</tr>
<tr>
<td id="21" onclick="colorChange(this, 21)">40</td>
<td id="22" onclick="colorChange(this, 22)">2</td>
<td id="23" onclick="colorChange(this, 23)">4</td>
<td id="24" onclick="colorChange(this, 24)">8</td>
<td id="25" onclick="colorChange(this, 25)">16</td>
</tr>
</table>
</body>
<script>
function colorChange(t, s){
let tdstyle = t.style.backgroundColor;
let hnum = s%5; // 아이디를 5로 나눈 나머지값
let wnum = parseInt((s-1)/5);
let heightBingoTrue = true;
let widthBingoTrue = true;
let leftBingoTrue=true;
let rightBingoTrue=true;
if(tdstyle == "red"){
t.style.backgroundColor = "white";
}else{
t.style.backgroundColor = "red";
}
// 대각선빙고여부 확인(왼)
for(let i=1;i<=25; i+=6){
if(document.getElementById(i).style.backgroundColor !="red"&&
document.getElementById(i).style.backgroundColor != "gold"){
leftBingoTrue=false;
}
}
//대각선 골드로 변경
if(leftBingoTrue){
leftBingoChange();
}
// 대각선빙고여부 확인(오)
for(let i=5;i<25; i+=4){
if(document.getElementById(i).style.backgroundColor !="red"&&
document.getElementById(i).style.backgroundColor != "gold"){
rightBingoTrue=false;
}
}
//대각선 골드로 변경
if(rightBingoTrue){
rightBingoChange();
}
//가로빙고여부 확인
for(let i = 0; i <= 25; i++){
if(parseInt(i / 5) == wnum){
if(document.getElementById(i+1).style.backgroundColor != "red"){
widthBingoTrue = false;
}
}
}
//가로줄 골드로 변경
if(widthBingoTrue){
widthBingoChange(wnum);
}
//세로빙고여부 확인
for(let i = 1; i <= 25; i++){
if(i % 5 == hnum){
if(document.getElementById(i).style.backgroundColor != "red"&&
document.getElementById(i).style.backgroundColor != "gold"){
heightBingoTrue = false;
}
}
}
//세로줄 골드로 변경
if(heightBingoTrue){
heightBingoChange(hnum);
}
}
// 가로줄 변경 함수
function widthBingoChange(wnum){
for(let i = 1; i <= 25; i++){
if(parseInt((i-1)/5) == wnum){
document.getElementById(i).style.backgroundColor = "gold";
}
}
}
// 세로줄 변경 함수
function heightBingoChange(hnum){
for(let i = 1; i <= 25; i++){
if(i % 5 == hnum){
document.getElementById(i).style.backgroundColor = "gold";
}
}
}
function leftBingoChange(){
for(let i=1; i<=25; i+=6){
document.getElementById(i).style.backgroundColor = "gold";
}
}
function rightBingoChange(){
for(let i=5; i<25; i+=4){
document.getElementById(i).style.backgroundColor = "gold";
}
}
</script>
</html>
'IT코딩공부!' 카테고리의 다른 글
#30 JS(history, 팝업창 띄우기!!) JSP공부하기 시작!!! (1) | 2023.05.12 |
---|---|
#29 JS(jquery 배우기) (0) | 2023.05.11 |
#27 JS( ATM기 만들어보기ex)함수사용, Event생성 (0) | 2023.05.08 |
#26 JS(중첩for문, 변수영역, 배열, 객체) (0) | 2023.05.04 |
#25 JS(조건,반복) 계산기2!!! (0) | 2023.05.03 |